Table of Contents
Ever feel like your code's a tangled mess? Like trying to find a matching sock in a black hole? That's where the concept of "calisthenics object" comes in. Think of it as a workout routine for your code, a set of rules to whip your object-oriented programming into shape. Jeff Bay came up with nine simple, yet powerful guidelines, and they're all about making your code easier to read, change, and test. We're not talking about getting your code to do push-ups, but rather about building a solid foundation for maintainable software. Ready to learn these coding exercises? We'll walk through each of the 9 rules of calisthenics object, show you how to actually use them, and explain why following them can save you headaches down the road.
9 Rules of Calisthenics Object
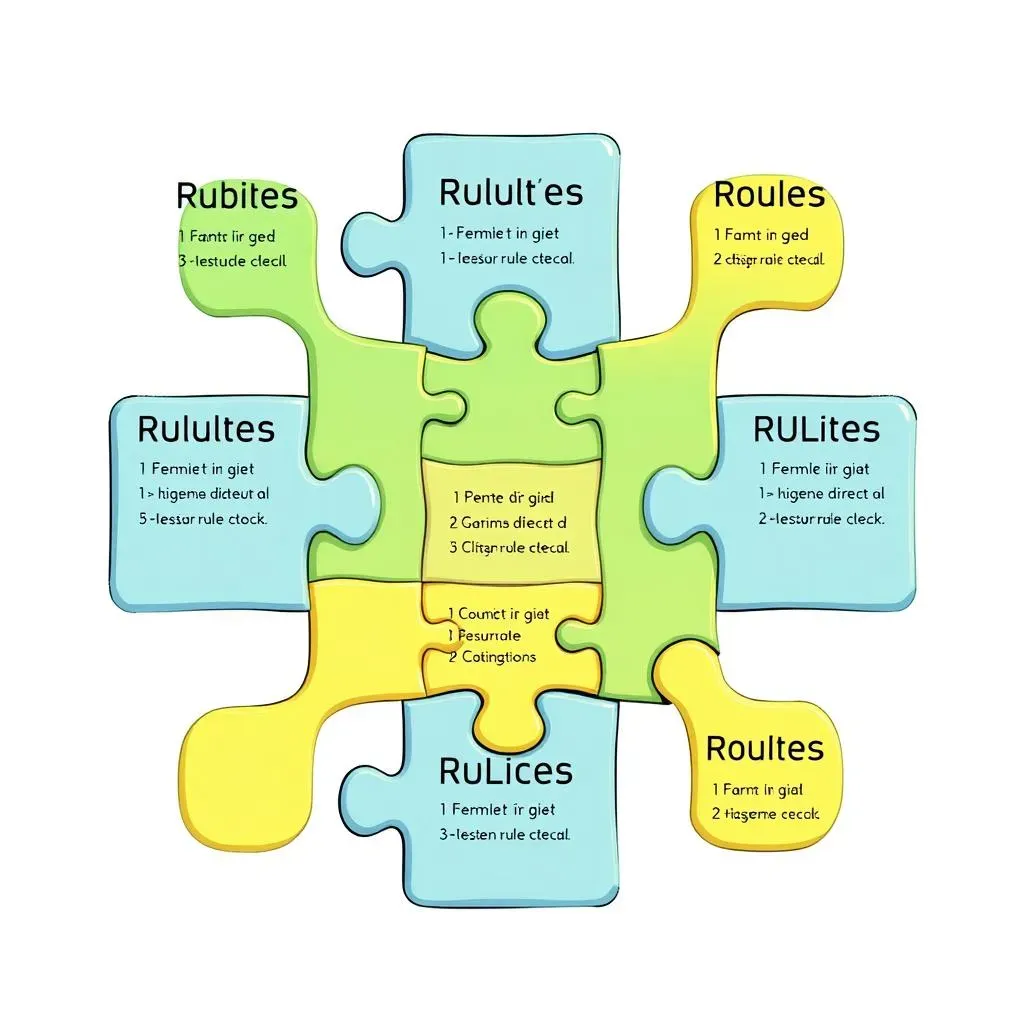
9 Rules of Calisthenics Object
One Level of Indentation Per Method
Imagine your code is like a set of Russian nesting dolls. You open one, and there's another inside, and another, and another. That's kind of what deep indentation looks like in code. It gets confusing fast! This first rule says to keep it shallow. Think of it like only opening one doll at a time. Each method, which is like a mini-program inside your bigger program, should only have one level of indentation. This makes it way easier to see what's going on without getting lost in a maze of code.
For example, instead of having an `if` statement inside another `if` statement inside a `for` loop, you'd try to find a simpler way to write it. It might mean breaking your code into smaller, more focused methods. It's like saying, instead of one long complicated instruction, give a few short, clear directions.
Don't Use the ELSE Keyword
Yeah, you read that right. No `else`! This might sound weird, but it actually pushes you to think more carefully about your conditions. The `else` keyword can sometimes be a crutch, leading to code that's harder to follow. Without it, you often end up with code that's more direct and easier to understand. Think of it like this: instead of saying "If it's raining, take an umbrella, otherwise don't," you'd say "If it's raining, take an umbrella." The "otherwise" is implied by the lack of the first condition.
How do you do this? Often, it involves using something called "guard clauses." These are like early exits from a method. If a certain condition isn't met, you just return or stop the method right there. This avoids the need for an `else` block to handle the other case. It makes your code flow more predictably.
Rule | Why It's Important |
---|---|
One Level of Indentation Per Method | Improves readability and reduces complexity. |
Don't Use the ELSE Keyword | Encourages clearer conditional logic and reduces nesting. |
Applying Calisthenics Object Principles
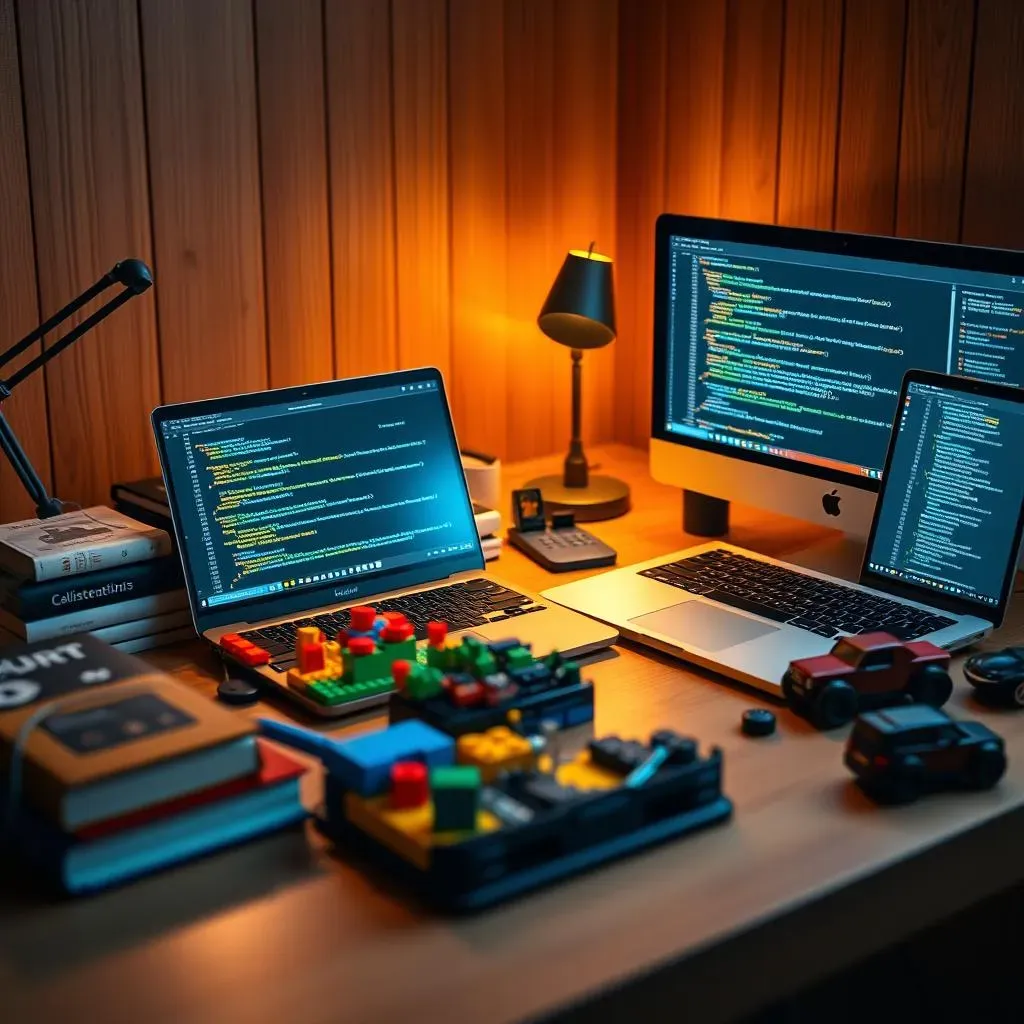
Applying Calisthenics Object Principles
Wrapping Primitives
Think of it like this: instead of just handing someone a loose Lego brick, you give them a whole little Lego kit. That Lego brick is like a primitive – a simple piece of data like a number or a word. The rule about wrapping primitives means you shouldn't just pass these basic bits around on their own. Instead, bundle them up into objects that give them context and behavior. For example, instead of having a plain old string for a customer's name, you might create a `CustomerName` object. This object can then have methods to validate the name or format it correctly. It keeps things organized and makes your code easier to understand.
I remember once working on a project where we had plain integers representing different currencies all over the place. It was a nightmare! Was `5` dollars or euros? We kept mixing them up. Wrapping those integers in `Dollar` and `Euro` objects would have saved us so much trouble. It's about giving those lonely pieces of data some friends and responsibilities.
First-Class Collections
Imagine you have a bunch of toy cars. Instead of just scattering them all over the floor, you put them in a toy box. That toy box is like a first-class collection. This rule says that if you have a collection of things, like a list of orders or a group of customers, that collection should be an object in its own right. Don't just use a generic list or array. Create a specific class for that collection, like an `OrderList` or a `CustomerGroup`. This lets you add behavior that's specific to that group of items. For instance, your `OrderList` could have a method to calculate the total value of all orders.
I've seen code where people just pass around lists of things, and then every time they need to do something with that list, they have to write the same logic over and over. Creating a first-class collection is like giving that list its own superpowers. It keeps the related logic together and makes your code much cleaner. It's like giving your toy cars their own garage with tools and a mechanic.
Principle | Real-World Analogy | Coding Benefit |
---|---|---|
Wrapping Primitives | Giving a Lego brick a kit | Provides context and behavior to simple data. |
First-Class Collections | Putting toy cars in a toy box | Encapsulates collection logic and improves organization. |
Benefits of Using Calisthenics Object
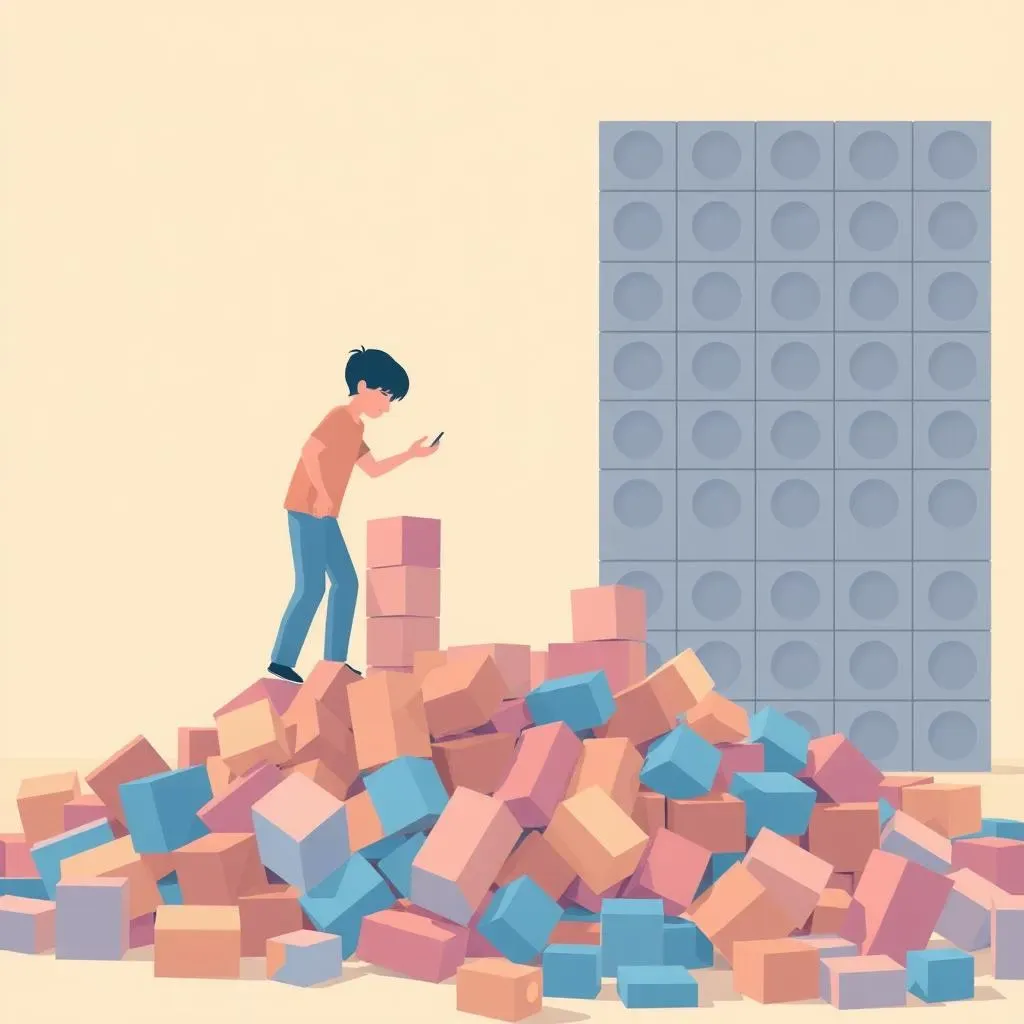
Benefits of Using Calisthenics Object
Alright, so why bother with all these rules? It might seem like extra work, like having to put your toys away in exactly the right spot. But trust me, it pays off. Think of it this way: would you rather build a fort with neatly stacked blocks or a jumbled pile? Calisthenics object makes your codebase that stack of neat blocks. It's way easier for you (or someone else) to come back later and add to it, fix it, or even just figure out what the heck is going on. Plus, when your code is well-organized, it's less likely to have hidden bugs, those annoying little gremlins that cause everything to crash at the worst possible time. It's like brushing your teeth – it might seem like a chore, but it saves you from bigger problems later. Here's a quick rundown:
Benefit | Why It Matters |
---|---|
Easier to Understand | You (and others) can quickly grasp the code's purpose. |
Less Bug-Prone | Clearer structure reduces the chance of errors. |
Easier to Change | Modifications are less risky and time-consuming. |